— For Beginners
In this Blog, we will mainly focus on the important concept of State and Hooks and how State Management can be achieved using React Hooks.
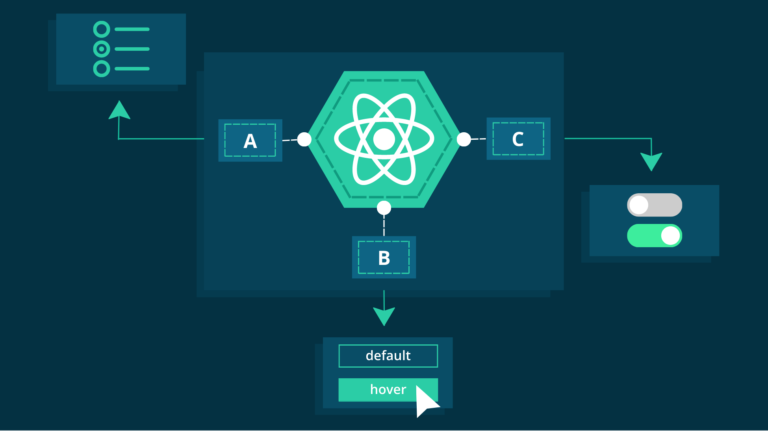
State Management with React Hooks
Before jumping into State Management, let’s first understand What is a State in React
Introduction to State in React
State in react allows us to change the data in an app. It is an object, in which we give key-value pairs for the different types of data that we want the app to track.
Over time, a component’s state may change, each time it re-renders. The rendering and behavior of the component are controlled by the changes to the state, which might occur in reaction to user input or system-generated events.
How do we Define, Use, and Modify the State Object?
Initializing State
First, the state object is initialized in the constructor as follows:
this.state = {name: “Alex”}
Changing State
For changing the state, we get a setState function from react that allows us to update the value of the state.
setState(updater, [callback])
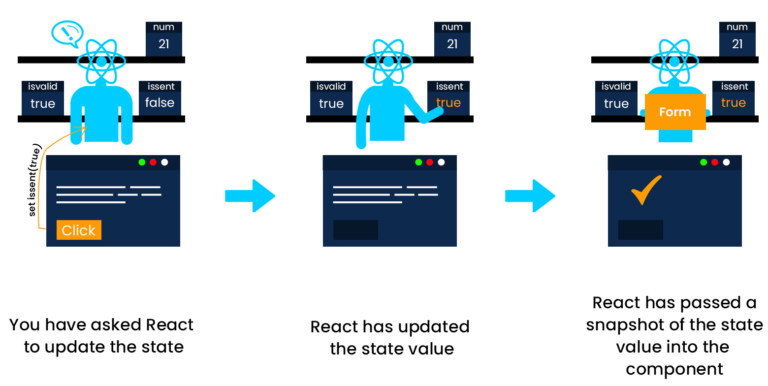
State Management with React Hooks
Example:
class Keyboard extends React.Component {
constructor(props) {
super(props);
// Defining States
this.state = {
brand: “A4Tech”,
color: “Black”,
model: “KK-3 Keyboard”,
available: true,
price: “20$”
};
}
// Updating the State
changeAvailabiliy = () => {
this.setState({available: !this.state.available});
}
render() {
return (
<div>
<h1>Keyboard On Sale!</h1>
/* Using States */
<p>
A {this.state.color} colored
{this.state.brand}-
{this.state.model}.
</p>
<p>Price: {this.state.price}</p>
<p>{this.state.available ? “Available” : “Unavailable”}</p>
<button
type=“button”
onClick={this.changeAvailabiliy}
>Change Availability</button>
</div>
);
}
}
So, whenever we want to change any state, we will update the state of that key and the component will re-render with the updated state.
Now that we have an understanding of State in React, let’s grab the concepts of React Hooks and how they help us in State Management.
Introduction to React Hooks?
The most interesting feature of Hooks introduced by React after v16.8. The good thing about Hooks is that they let us use the state and other features of React in a functional component without even writing a class
State Management with React Hooks
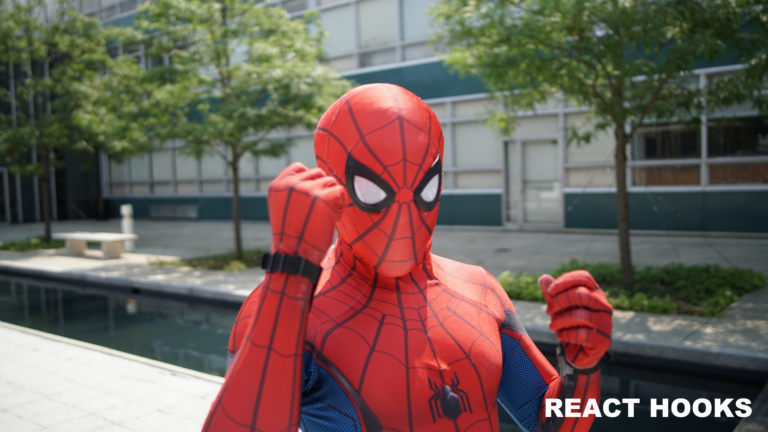
React Hook
Now, let’s see 3 important rules for hooks to remember:
Hooks can only be called inside React functional components.
Hooks can only be called at the top level of a component.
Hooks cannot be conditional
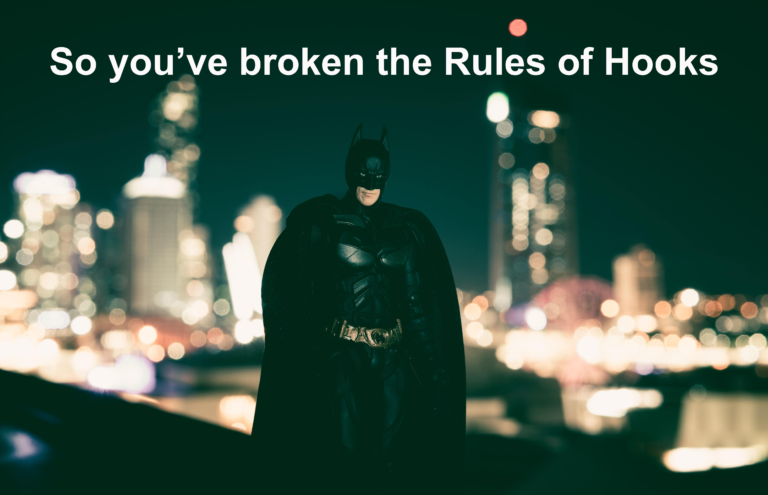
Don’t break the rules
Don’t break the rules, right? Now let’s grab the process of state management with React Hooks.
State Management with React Hooks
React offers multiple Hooks for State Management. We will learn how to set state using the useState and useEffect hooks.
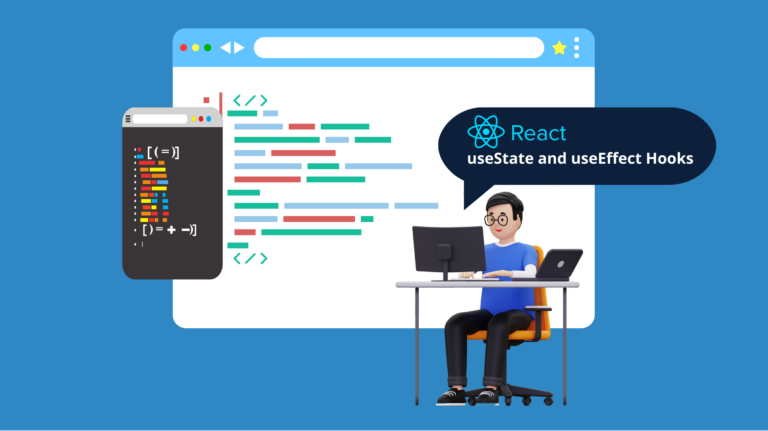
React useState and useEffect Hooks.
Using useState Hook
The React useState Hook allows us to track state in a function component. We have another hook used in place of useState hook i.e useReducer hook, but there is a slight difference between both.
The useState hook is effective when we set any value without referencing the current state, whereas useReducer is helpful when we need to reference a prior value or when we have several actions that call for complicated data manipulations.
import { useState } from “react”;
Then, we initialize it by calling useState in the function component. It accepts the initial state and returns 2 values:
The current state.
A function that updates the state.
import { useState } from “react”;
function myAge() {
const [age, setAge] = useState(1);
}
To read the state, we can now include our state anywhere in the component.
function MyAge() {
const [age, setAge] = useState(1);
return <h1>I am {age}y Old!</h1>
}
const root = ReactDOM.createRoot(document.getElementById(‘root’));
root.render(<My Age/>);
We use state updater function to update our state
function MyAge() {
const [age, setAge] = useState(1);
return (
<>
<h1>I am {age}y Old!</h1>
<button
type=”button”
onClick={() => setAge(21)}
>21</button>
</>
)
}
Using useEffect Hook
We use useEffect Hook to perform side effects in the components. A few examples of these side effects are:
directly updating the DOM
fetching data
timers etc.
Using useState Hook
We use useEffect Hook to perform side effects in the components. A few examples of these side effects are:
directly updating the DOM
fetching data
timers etc.
Method:
useEffect accepts 2 arguments:
The 1st argument will accept the function.
The 2nd argument is optional for the dependency.
useEffect(<function>, <dependency>)
useEffect runs on every render. We should include the second parameter always if we want to render it with some dependency.
We first need to import it into our component.
import { useState, useEffect } from “react”;
There are 3 ways to use the useEffect hook.
No dependency passed:
When we will pass no parameter in the second argument, then the code inside the useEffect will run on every render.
useEffect(() => {
//Runs on every render
});
An empty array:
When we will pass an empty array in the second argument, then the code inside the useEffect will run only on the first render.
useEffect(() => {
//Runs only on the first render
}, []);
Props or state values:
When we will pass any dependency in the second argument, then the code inside the useEffect will run on the first render and every state change of that dependency.
useEffect(() => {
//Runs on the first render
//And any time any dependency value changes
}, [prop, state]);
Example:
import { useState, useEffect } from “react”;
import ReactDOM from “react-dom/client”;
function CurrentLevel() {
const [level, setLevel] = useState(0);
const [score, setScore] = useState(0);
useEffect(() => {
setScore(() => level* 2);
}, [level]); // <– It will effect on every state change of “count”
return (
<>
<p>Level: {level}</p>
<button onClick={() => setLevel((l) => l+ 1)}>+</button>
<p>Score: {score}</p>
</>
);
}
const root = ReactDOM.createRoot(document.getElementById(‘root’));
root.render(<Timer />);
In the above example, we used the useState and useEffect hooks in a single function. useState hook keeps the states of level and score, while useEffect hook updates the value of the score on each level change. This is how we can manage States in React.
Conclusion
Congratulations! You have learnt the basics of State Management in React that is one of the important features to understand.States improved the performance of the app and provide ease to the developers while working with the app.
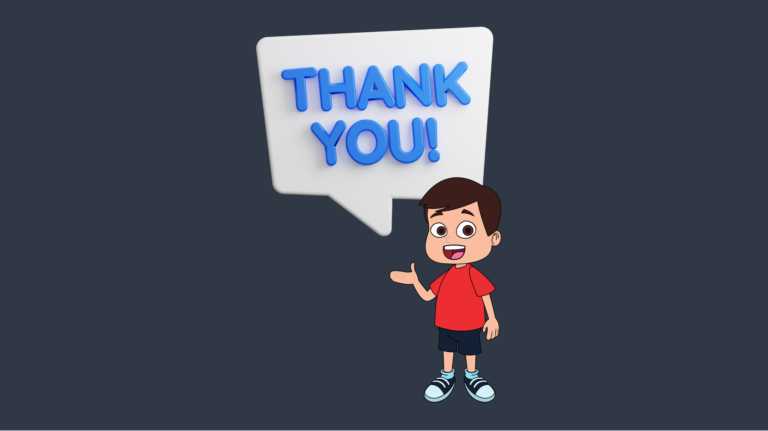
In the end, I want to say a huge Thanks for reading this blog! You can explore more Hooks such as useReducer, useMemo, and useContext and more ways of state management from their official website — https://reactjs.org/docs/hooks-intro.html.
If you want to connect with us, stay tuned for our recent blogs. You can also contact us at: https://bitsol.tech/contact/
Happy Learning!